Wandering Off
I tend to wander. Be it in downtown Beijing or just reading a book.
I'm still reading Java for Artists. I made it a couple of pages in several hours. Here's a rundown of the journey.
Chapter 3 talks about the Spiral Development Cycle.
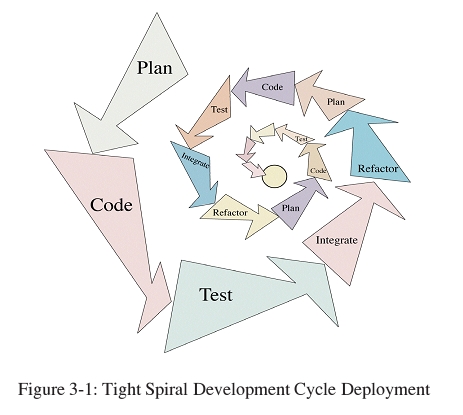
The same 3 steps (plan, code, test) are repeated to build and maintain forward progress. And although the code and testing have peristent artifacts (the code itself), the plan steps are layed out in tables in the book. Somewhere along the line I decided I wanted to be abkle to do everything in text files (Starting from scratch). I'd like continue this process after I'm done with the book, but how can I create structured data in a text file? XML. Damn it! Now I have to go read up on XML again. How many times does this make now? Use it or lose it. A google search on XML turns up a favorite site of mine (w3schools.com). Of Course, I can't just read about XML (since alone it won't really meet my needs). This leads me to tutorials on XML, XPath, XSLT and XSL-FO (that's a new one to me).
I probably shouldn't be watching tv while I'm doing all of this...
So now I'm working on the XML and XSL that I hope will make up my plan list. Java? Yeah, I'll get back to that eventually. At the moment I can't even get the XSL to properly transform the XML. I am Clearly a masochist.
Here's the xml to this point:
And the xsl:
After hours of trial and error. I find that removing the xmlns attribute from the dev_plan element makes everything work. Do I go and figure out why it was breaking things or do I move on with what I should be doing? ROFLOL
Before I get back to figuring out what went wrong with the xml, I should mention that I took another side trip to figure out / remember how display code on a web page. Doing a google search for displaying HTML code brought me to the xmp tag which I'd never heard of, but didn't work for me (meaning, just throwing it around the raw code didn't work). Finally I found an online tool to Display HTML sample code. Thank You Christopher Brewer ^_^
After being left unfulfilled by the explaination of XML Namespaces at w3schools (first time for everything), I found this article at xml.com. Interesting, but I still don't quite get it. Several variations on the xml still result in it not making it through the xsl. I know how to make it work, but I want to understand what's going on when it's not working. After reading Ronald Bourret's XML Namespaces FAQ I understand namespaces themselves a bit more, and get the impression that mine is declared properly, but not why the xsl "breaks".
After doing a search for "XML Namespaces breaks xslt xsl transform" I found a link to a thread that explains the problem and the solution (I didn't want). It looks like my options are to remove the default namespace from my xml doc OR prefix everything in both documents.
After a few more unsuccessful tests and in the interst of continuing my java studies I will remove the default xmlns attribute. Fortunitely this R&D won't be wasted as I will be visiting XML in a later study session.
I'm still reading Java for Artists. I made it a couple of pages in several hours. Here's a rundown of the journey.
Chapter 3 talks about the Spiral Development Cycle.
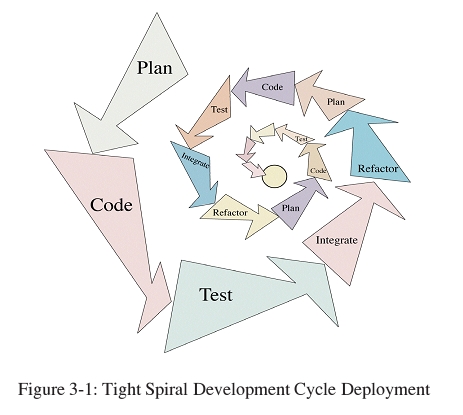
The same 3 steps (plan, code, test) are repeated to build and maintain forward progress. And although the code and testing have peristent artifacts (the code itself), the plan steps are layed out in tables in the book. Somewhere along the line I decided I wanted to be abkle to do everything in text files (Starting from scratch). I'd like continue this process after I'm done with the book, but how can I create structured data in a text file? XML. Damn it! Now I have to go read up on XML again. How many times does this make now? Use it or lose it. A google search on XML turns up a favorite site of mine (w3schools.com). Of Course, I can't just read about XML (since alone it won't really meet my needs). This leads me to tutorials on XML, XPath, XSLT and XSL-FO (that's a new one to me).
I probably shouldn't be watching tv while I'm doing all of this...
So now I'm working on the XML and XSL that I hope will make up my plan list. Java? Yeah, I'll get back to that eventually. At the moment I can't even get the XSL to properly transform the XML. I am Clearly a masochist.
Here's the xml to this point:
<?xml version="1.0" encoding="ISO-8859-1"?>
<?xml-stylesheet type="text/xsl" href="RobotRat.xsl"?>
<dev_plan xmlns="http://brian.donoho.com/xml/">
<name>RobotRat</name>
<prog_lang>Java</prog_lang>
<step id="1" phase="1">
<design_consideration>
Program Structure
</design_consideration>
<design_decision>
One class will contain all the functionality
</design_decision>
</step>
<step id="2" phase="1">
<design_consideration>
Creating the Java application class
</design_consideration>
<design_decision>
The class name will be RobotRat. It will contain a “public static void main(String[] args){ }” method.
</design_decision>
</step>
<step id="3" phase="1">
<design_consideration>
constructor method
</design_consideration>
<design_decision>
Write a class constructor that will print a short message to the screen when a RobotRat object is created.
</design_decision>
</step>
<step id="" phase="">
<design_consideration>
</design_consideration>
<design_decision>
</design_decision>
</step>
<step id="" phase="">
<design_consideration>
</design_consideration>
<design_decision>
</design_decision>
</step>
</dev_plan>
And the xsl:
<?xml version="1.0" encoding="ISO-8859-1"?>
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform">
<xsl:template match="/">
<html>
<body>
<h2><xsl:value-of select="dev_plan/name" /> Dev Plan</h2>
<table border="1">
<tr>
<th>Check-Off</th>
<th>Design Consideration</th>
<th>Design Decision</th>
</tr>
<tr>
<td></td>
<td></td>
<td></td>
</tr>
<xsl:for-each select="dev_plan/step">
<tr>
<td></td>
<td><xsl:value-of select="design_consideration" /></td>
<td><xsl:value-of select="design_decision" /></td>
</tr>
</xsl:for-each>
</table>
</body>
</html>
</xsl:template>
</xsl:stylesheet>
After hours of trial and error. I find that removing the xmlns attribute from the dev_plan element makes everything work. Do I go and figure out why it was breaking things or do I move on with what I should be doing? ROFLOL
Before I get back to figuring out what went wrong with the xml, I should mention that I took another side trip to figure out / remember how display code on a web page. Doing a google search for displaying HTML code brought me to the xmp tag which I'd never heard of, but didn't work for me (meaning, just throwing it around the raw code didn't work). Finally I found an online tool to Display HTML sample code. Thank You Christopher Brewer ^_^
After being left unfulfilled by the explaination of XML Namespaces at w3schools (first time for everything), I found this article at xml.com. Interesting, but I still don't quite get it. Several variations on the xml still result in it not making it through the xsl. I know how to make it work, but I want to understand what's going on when it's not working. After reading Ronald Bourret's XML Namespaces FAQ I understand namespaces themselves a bit more, and get the impression that mine is declared properly, but not why the xsl "breaks".
After doing a search for "XML Namespaces breaks xslt xsl transform" I found a link to a thread that explains the problem and the solution (I didn't want). It looks like my options are to remove the default namespace from my xml doc OR prefix everything in both documents.
After a few more unsuccessful tests and in the interst of continuing my java studies I will remove the default xmlns attribute. Fortunitely this R&D won't be wasted as I will be visiting XML in a later study session.
Labels: java, productivity, programming